Using functions and adding logic to your prototypes is straightforward in Origami, but a little different to what you might expect if you come from a programming background. If you haven’t touched code before, feel free to skip forward to Adding Logic.
Patches and functions
The basic building blocks in Origami are called patches. They are similar to functions as far as they take data input(s), perform an action on it, and produce a result.
function name(input1, input2, input3) {
code to be outputted
}
Origami patches work in the same way; taking a single or multiple input(s), performing an action, and producing an output — albeit using a slightly different format.
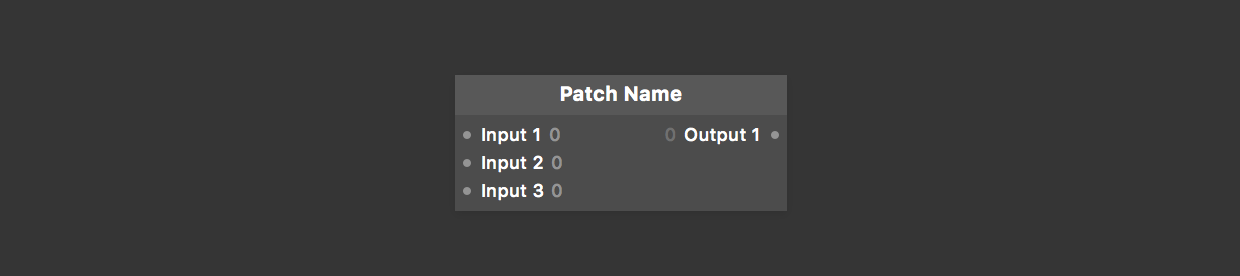
What makes Origami unique is the suite of pre-made patches that allow you to listen for various types of interaction, create natural animations, manipulate layer properties, and more. Just like functions in code, you can also create your own patches.
Inputs and outputs
We are using an example of converting Fahrenheit to Celsius. Fahrenheit being our Input value, and Celsius being our returned value, or Output.
In programming languages such as JavaScript, this calculation would look something like this:
function fahrenheitToCelsius(fahrenheit) {
return (5/9) * (fahrenheit-32);
}
var text = fahrenheitToCelsius(86);
Calculations done in patches are hidden from view by default. The patch simply presents the published Input(s) and Output(s).
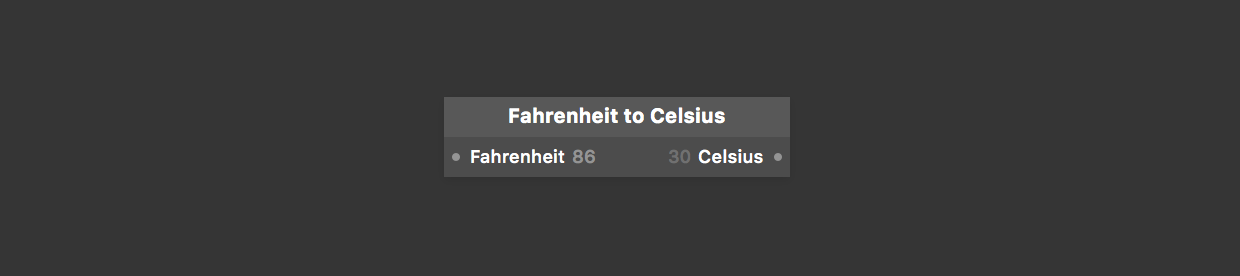
Entering this custom-made patch through Patch > Enter Patch Group ⌘↓ reveals the same calculations as in code.
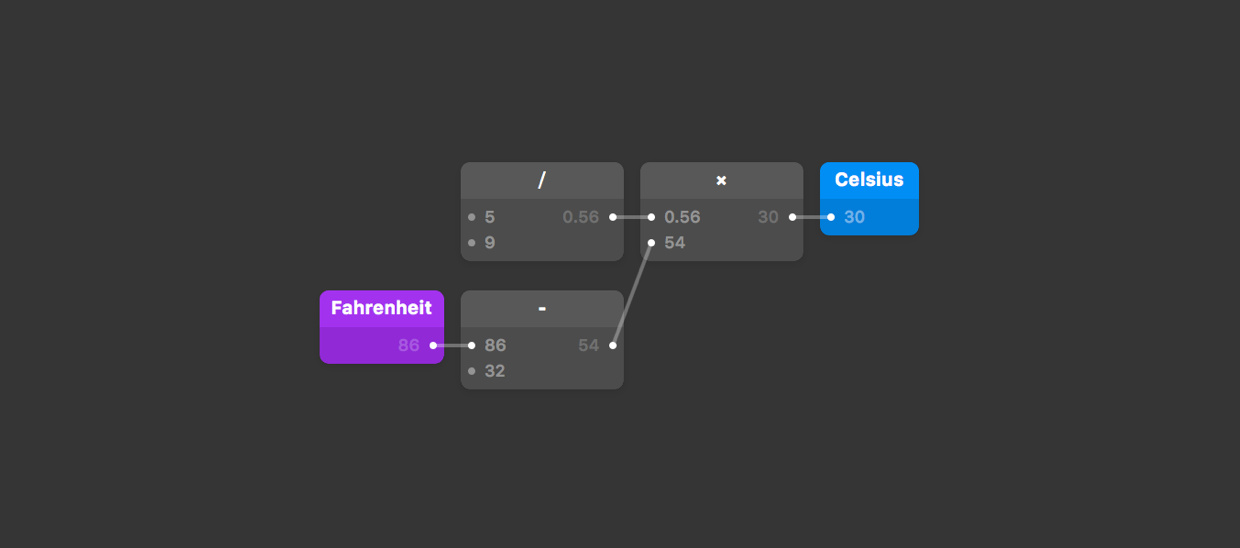
One important thing to take note of is the order of calculations. Code usually follows the traditional order of mathematical operations; prioritizing some operators (/
,*
) over others (+
, -
). Origami simply calculates from the order in which the patches are connected.
Multiple outputs
Returning multiple outputs is easy in Origami. This means patches can be used beyond what functions are typically used for. As an example, here is our temperature calculating patch going beyond Fahrenheit to Celsius:
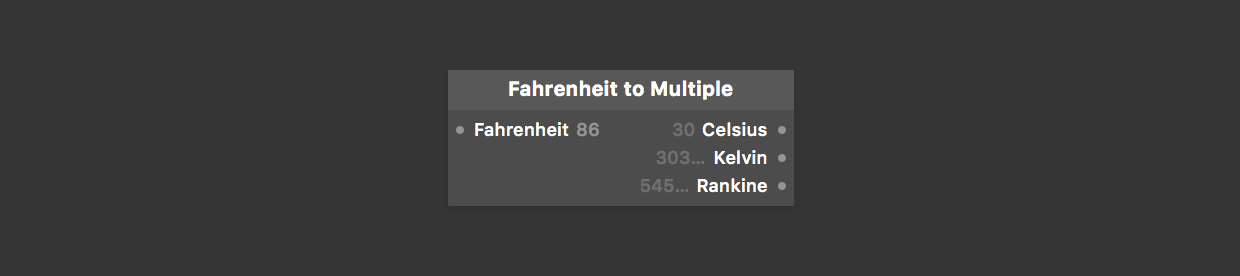
Values calculated inside the patch simply need to be published as outputs:
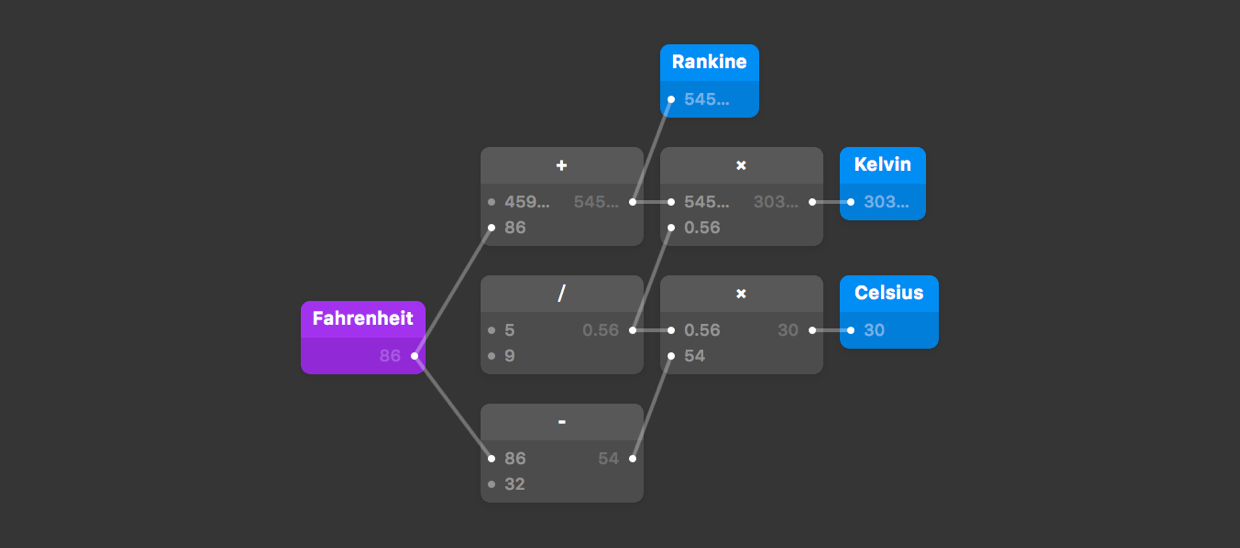
Logic and conditionals
Logic in code, called conditionals, is a little different from the way Origami handles logic. Code usually runs linearly from top to bottom, and requires calculations to be done before moving to the next line.
In Origami’s visually-focused Patch Editor, information flows from left to right (including logical operations), depending on what is being interacted with and/or triggered. For that reason, you won’t find patches analogous to if
, else
, else if
, or while
found in code. Instead, information passed through patches (usually from an interaction) will only continue to flow unless the comparison is false.
Doing math
Math in Origami is largely the same as arithmetic operators in code. Origami has patches for most standard operators.
3+2
3-2
3*2
3/2
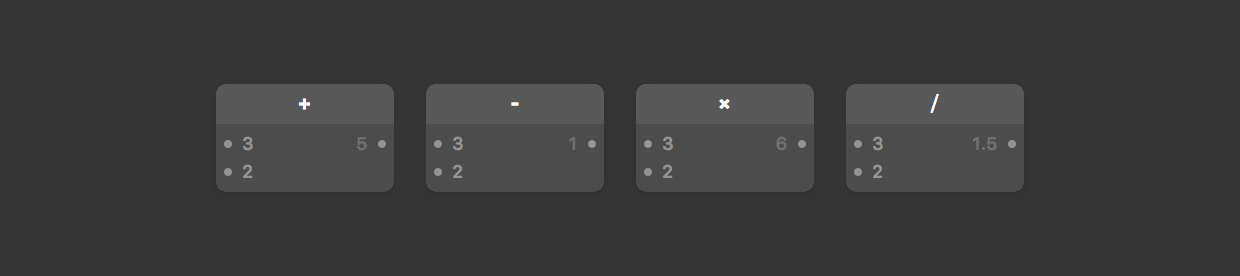
Comparing items
Origami is able to take values and output a true or false boolean value, similar to comparison operators in code.
3>2
3<2
3==2
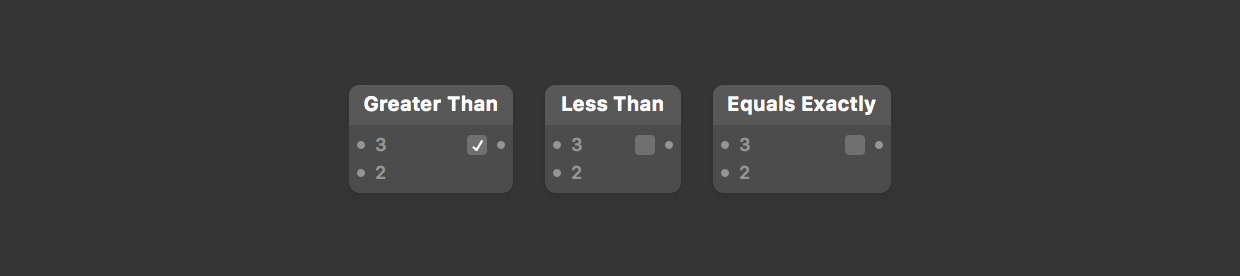
Comparing with logic
This is where things start to diverge. Origami has built-in patches which allow for common logic to be done quickly and easily. For example:
3>=2
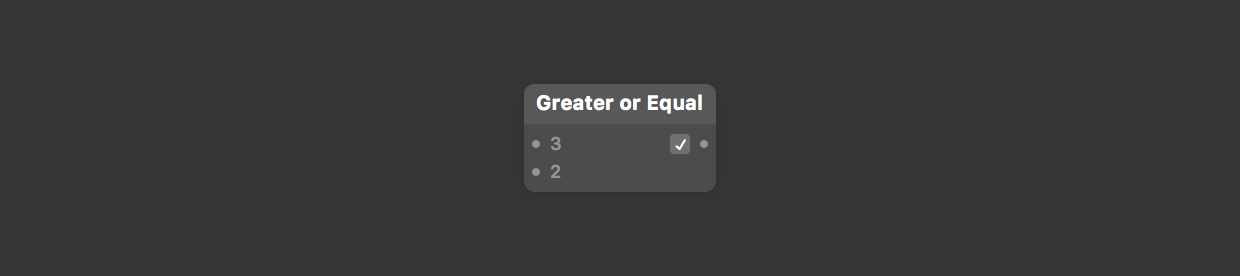
In code, a logical operator is usually paired with a comparison operator (as shown above). Origami makes it easy to make comparisons with dedicated patches for common logic:
&&
!
||
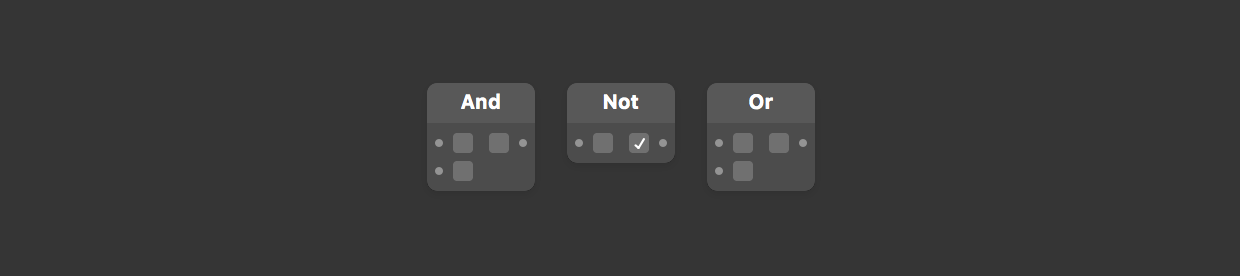
These patches are useful for checking conditionals—similar to if
or else
. Take an example of checking to see if a calculation is true:
if (3>2 && (2==3||2<3) && 2!=>3) {
code to be executed if true
}
Origami takes the information flowing left to right (analogous to 3>2 && (2==3||2<3) && 2!=>3
above) and outputs either true or false, represented by the output on the And patch:
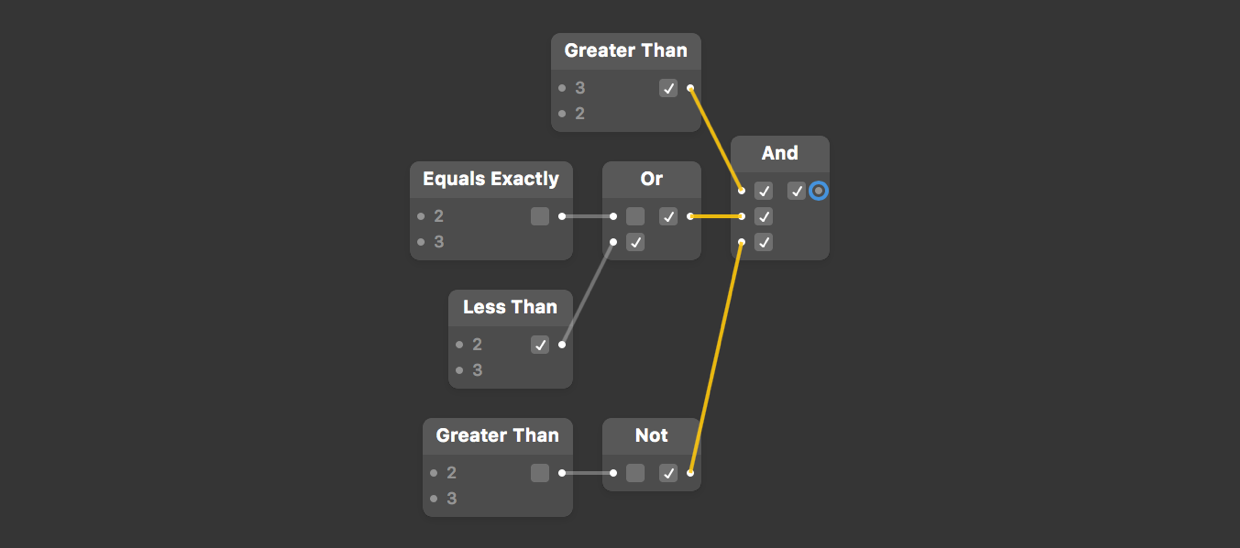
Chaining multiple calculations can become complex and difficult to manage in code. This task becomes intuitive and flexible when built in Origami.